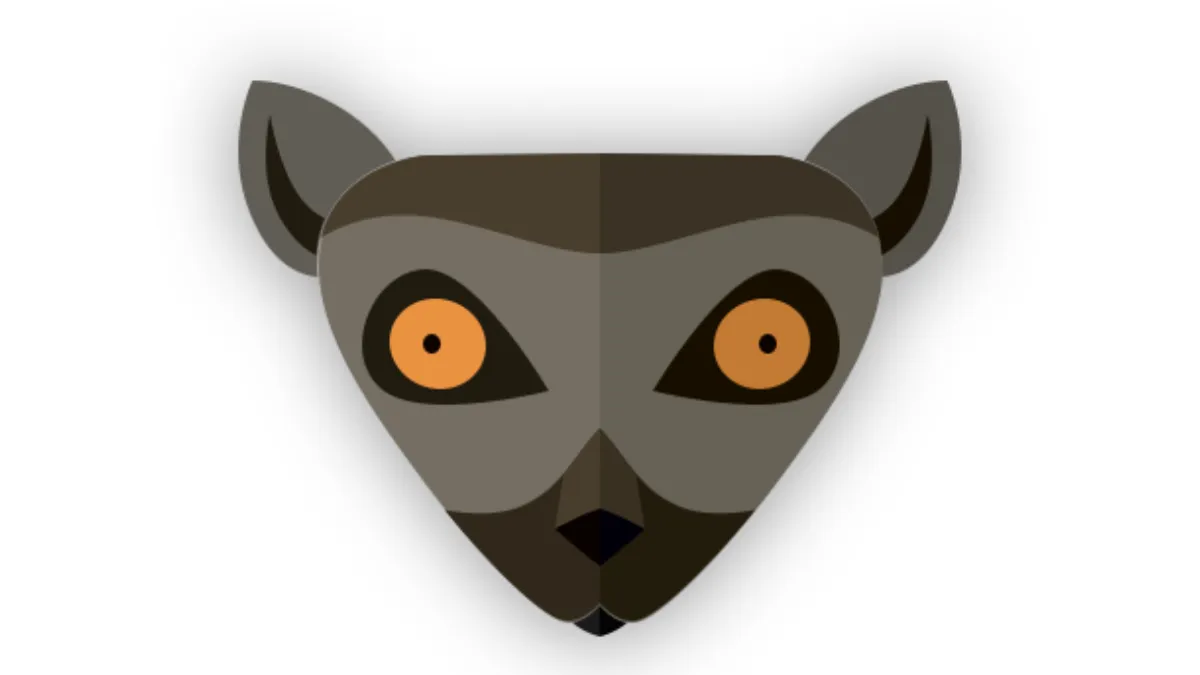
Inhaltsverzeichnis
Introduction BackstopJS
BackstopJS is a powerful open source tool for automated visual regression testing of web applications. It enables developers to track and compare visual changes in their web applications over time by creating and analysing screenshots.
Installation and setup of BackstopJS
Install BackstopJS globally via npm:
npm install -g backstopjs
For a local installation in your project:
npm install --save-dev backstopjs
Initialise a new BackstopJS project:
backstop init
Configuration and customisation of BackstopJS
The flexibility of BackstopJS allows for detailed customisation to meet the needs of your project. Here are some advanced configuration options and customisations:
Viewport definitions
Define multiple viewports for responsive tests:
"viewports": [
{
"label": "phone",
"width": 320,
"height": 480
},
{
"label": "tablet",
"width": 1024,
"height": 768
},
{
"label": "desktop",
"width": 1920,
"height": 1080
}
]
Scenario options
Configure detailed scenarios:
"scenarios": [
{
"label": "Homepage mit Interaktion",
"url": "https://example.com",
"delay": 500,
"clickSelectors": [
".menu-toggle",
".submenu-item"
],
"hoverSelector": ".hover-effect",
"postInteractionWait": 1000,
"selectors": [
"header",
"main",
"footer"
],
"selectorExpansion": true,
"expect": 0,
"misMatchThreshold": 0.1
}
]
Custom scripts
Create custom scripts for complex interactions:
// onBefore.js
module.exports = async (page, scenario, vp) => {
await page.evaluate(() => {
// Deaktivieren von Animationen
document.body.classList.add('no-animations');
});
};
// onReady.js
module.exports = async (page, scenario, vp) => {
await page.evaluate(() => {
// Benutzerdefinierte Logik nach dem Laden der Seite
});
};
Engine options
Configure the engine used (e.g. Puppeteer):
"engineOptions": {
"args": ["--no-sandbox"],
"ignoreHTTPSErrors": true,
"headless": true
}
Report customisations
Customise the report generation:
"report": ["browser", "CI"],
"compareOptions": {
"output": {
"errorColor": {
"red": 255,
"green": 0,
"blue": 255
},
"errorType": "movement",
"largeImageThreshold": 1200,
"useCrossOrigin": false,
"outputDiff": true
},
"scaleToSameSize": true,
"ignore": "antialiasing"
}
Environment variables
Use environment variables for flexible configurations:
"scenarios": [
{
"label": "Dynamische URL",
"url": "${TEST_URL}",
"readySelector": "#content"
}
]
Set the environment variable when executing:
TEST_URL=https://staging.example.com backstop test
Advanced selectors
Use CSS and XPath selectors for precise tests:
"scenarios": [
{
"label": "Komplexe Selektoren",
"url": "https://example.com",
"selectors": [
"document",
{"selector": "//button[contains(@class, 'submit')]", "type": "xpath"},
{"selector": ".dynamic-content", "type": "css"}
]
}
]
File comparisons
Configure the comparison of files instead of URLs:
"scenarios": [
{
"label": "Lokale HTML-Datei",
"url": "file:///path/to/local/file.html",
"referenceUrl": "file:///path/to/reference/file.html"
}
]
Debugging options
Activate debug options for more detailed information:
"debug": true,
"debugWindow": true
Customised comparison methods
Implement customised comparison logic:
// customCompare.js
module.exports = async function(page, scenario, viewport, isReference, browserContext) {
// Benutzerdefinierte Vergleichslogik hier
return { misMatchPercentage: 0 }; // Beispielrückgabe
};
Use the customised comparison method in the configuration:
"scenarios": [
{
"label": "Benutzerdefinierter Vergleich",
"url": "https://example.com",
"customCompare": "customCompare.js"
}
]
These advanced configuration options and customisations allow you to tailor BackstopJS precisely to the requirements of your project. Experiment with these settings to create the most effective visual regression tests for your web application.
Use of BackstopJS
Basic commands
1. perform tests:
backstop test
2. Update reference screenshots:
backstop approve
3. Show report
backstop openReport
Integration in CI/CD-Pipelines
BackstopJS can be easily integrated into CI/CD pipelines. Here is an example for GitLab CI:
visual_regression_tests:
image: node:latest
stage: test
script:
- npm install -g backstopjs
- backstop test
artifacts:
paths:
- backstop_data
Tips and best practices BackstopJS
- Start with a few critical scenarios and expand step by step.
- Use meaningful labels for your scenarios.
- Adjust the
misMatchThreshold
to reduce false positives. - Use Docker for consistent results across different environments.
- Regularly check your reference screenshots and update them if you intend to make changes.
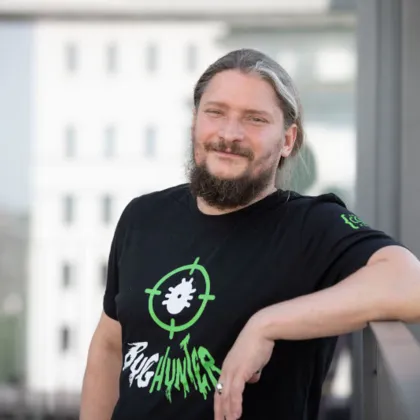
Reach our PHP Consultant specialists
We are experts in PHP and help you to master your digital challenges. Our experienced team supports you with PHP updates, PHP refactoring and advises you remotely on all questions relating to PHP. With our fully automated CI/CD deployments and a robust Docker infrastructure, we take your PHP projects to the next level. Rely on our expertise for reliable and scalable PHP solutions.
Frequently asked questions (FAQ)
How does BackstopJS handle dynamic content?
Use readySelector, readyEvent or delay to ensure that dynamic content is loaded before screenshots are created.
Can I use BackstopJS with different browsers?
Yes, BackstopJS supports various engines such as Puppeteer, Playwright and Chromy.
How can I exclude certain areas from the comparison?
Use hideSelectors or removeSelectors in your scenario configurations.
Does BackstopJS support responsive designs?
Yes, you can define multiple viewports in your configuration to test responsive designs.
How do I deal with animations?
Use postInteractionWait or custom scripts to wait or disable animations.
Can I integrate BackstopJS into my existing tests?
Yes, BackstopJS can be integrated into your existing Node.js tests as an npm module.
How do I handle pages that require authentication?
Use custom scripts or the cookiePath option to set authentication data.
Can I use BackstopJS for A/B tests?
Yes, you can define and compare different scenarios for A and B variants.
How do I optimise performance in many scenarios?
Use the asyncCaptureLimit and asyncCompareLimit options to control parallelism.
Does BackstopJS support email testing?
Yes, you can use BackstopJS to test HTML email templates by rendering them as static HTML pages.
Conclusion
BackstopJS is an indispensable tool for developers and QA teams who want to recognise and fix visual regressions in their web projects at an early stage. By integrating it into the development process and CI/CD pipelines, you can ensure that visual changes are always intentional and consistent. Try BackstopJS and improve the quality assurance of your web projects today!