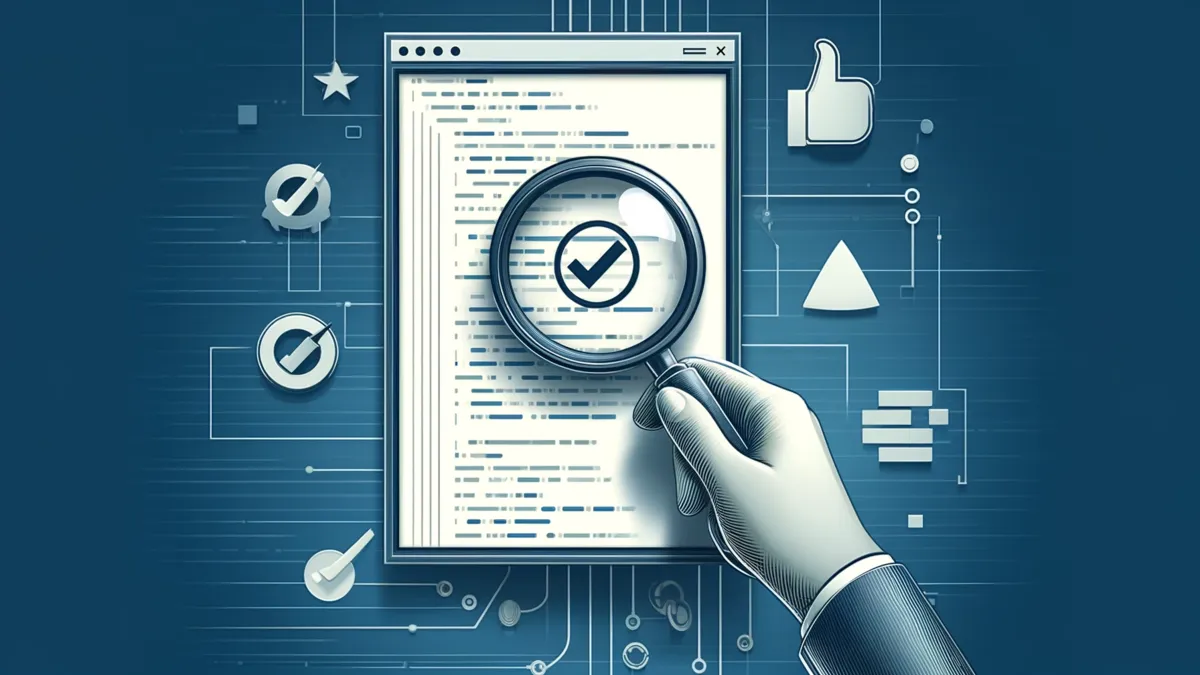
Table of contents
Easy Coding Standard: Automated code formatting in PHP
The Easy Coding Standard (ECS) is a powerful open source tool that helps you to automatically format and optimise your PHP code. By using predefined rules and conventions, ECS ensures a standardised and clean code style in your projects. In this article, you will learn how to integrate ECS into your PHP development environment and benefit from its advantages.
Installation and setup
To use ECS in your project, you must first ensure that you have Composer installed. You can then simply install ECS using the following command:
composer require --dev symplify/easy-coding-standard
After successful installation, you will find the file ecs.php in the main directory of your project, where you can make the configuration.
Configuration and customisation
The ecs.php file allows you to define the rules and conventions that ECS should apply to your code. Here you can choose from a variety of predefined sets, such as PSR-12 or Symfony Coding Standards. However, you also have the option of defining your own rules or adapting existing ones.
An example of a simple configuration:
use Symplify\EasyCodingStandard\Config\ECSConfig;
use PhpCsFixer\Fixer\ArrayNotation\ArraySyntaxFixer;
return static function (ECSConfig $ecsConfig): void {
$ecsConfig->paths([__DIR__ . '/src', __DIR__ . '/tests']);
$ecsConfig->rules([
ArraySyntaxFixer::class => ['syntax' => 'short'],
]);
};
In this example, we specify that ECS should check the src and tests directories. We also define a rule that enforces the short array syntax.
Use of ECS
Once you have installed and configured ECS, you can simply run it from the command line:
vendor/bin/ecs check
This command checks your code against the defined rules and gives you an overview of the problems found. With the additional --fix flag, you can instruct ECS to make the changes automatically:
vendor/bin/ecs check --fix
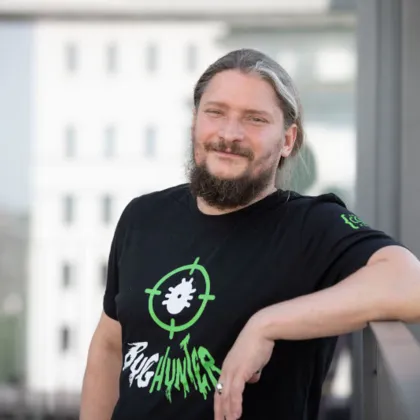
Reach our PHP Consultant specialists
We are experts in PHP and help you to master your digital challenges. Our experienced team supports you with PHP updates, PHP refactoring and advises you remotely on all questions relating to PHP. With our fully automated CI/CD deployments and a robust Docker infrastructure, we take your PHP projects to the next level. Rely on our expertise for reliable and scalable PHP solutions.
Best Practices
It is advisable to run ECS regularly to ensure that your code always complies with the desired conventions. You can run it manually or integrate it into your CI/CD pipeline.
Integration in CI/CD-Pipelines
To integrate ECS into your CI/CD pipeline, you need to make sure that the necessary dependencies are installed and the ecs check command is executed. Here is an example of a Github Actions workflow file:
name: CI
on: [push]
jobs:
ecs-check:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install dependencies
run: composer install --prefer-dist --no-progress --no-suggest
- name: Run ECS check
run: vendor/bin/ecs check
This workflow performs the ECS check for each push process and fails if violations of the defined rules are found.
Friendly asked questions
Can I apply ECS only to certain files or directories? A: Yes, you can use the ecs.php
configuration file to specify which paths are to be checked. For example: $ecsConfig->paths([__DIR__ . '/src', __DIR__ . '/tests']);
How can I exclude certain rules from the check?
In the configuration file, you can explicitly exclude rules by listing them in the skip section. For example: $ecsConfig->skip([ArraySyntaxFixer::class]);
Can I define my own rules?
Yes, you can create customised rules by implementing your own fixer classes and registering them in the configuration.
Does ECS support languages other than PHP?
No, ECS is specifically designed for PHP. There are similar tools for other languages, such as ESLint for JavaScript or Rubocop for Ruby.
Can I integrate ECS with PHPStorm?
Yes, there is a PHPStorm plugin that enables the integration of ECS into the IDE.
How can I ensure that my entire team uses the same code standards?
You can check the ecs.php configuration file into your project and make it part of your version control. This way, every developer automatically uses the same settings.
Can I customise ECS to comply with specific code standards of my company or project?
Yes, you can enforce company or project-specific standards by adapting the rules in the configuration file.
How often should I run ECS in my project?
It is advisable to run ECS regularly, e.g. with every commit or as part of your CI/CD pipeline. This allows you to recognise and rectify deviations at an early stage.
Does the use of ECS slow down my development process?
No, ECS is designed to work quickly and efficiently. Automatic code formatting even saves time in the long run, as you don't have to worry about style issues manually.
Can I also introduce ECS in existing projects?
Yes, you can add ECS to an existing project at any time. However, please note that the initial execution may make many changes to your code to adapt it to the defined standards.
Curious insights into the world of software development
The world of software development is full of idiosyncrasies, running gags and controversial discussions that are often difficult for outsiders to understand. Using code examples, memes and comics, this picture gallery provides entertaining insights into the everyday life of developers and the topics that prevail there.
Whether it's the eternal argument between tabs and spaces in indentation, the importance of clean formatting or the excesses of complex code constructs - here you will find amusing examples that get to the heart of many a coding discussion.
The images show in a humorous and sometimes exaggerated way how much developers are concerned with the details of their craft and how emotionally seemingly minor issues are discussed. At the same time, they emphasise the importance of standards, readability and maintainability in software development - even if the road to achieving this can sometimes be rocky.
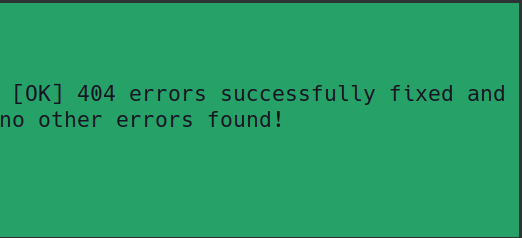
Linux Terminal Output: [OK] 404 errors successfully fixed and no other errors found!
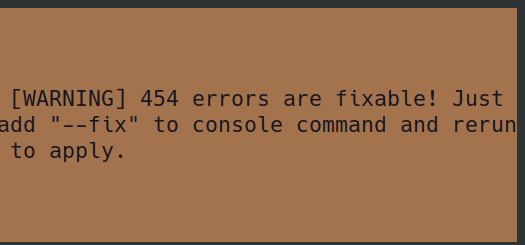
Linux Terminal output: [WARNING] 454 errors are fixable! Just add "--fix" to console command and rerun to apply.
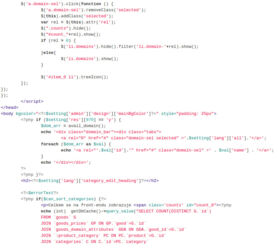
Coding Standards Medium
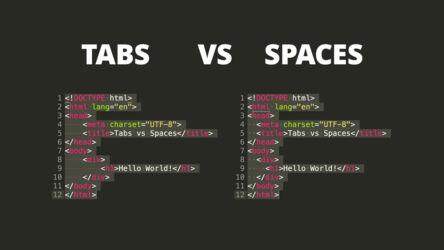
Tabs vsn Spaces von Doe Anderson
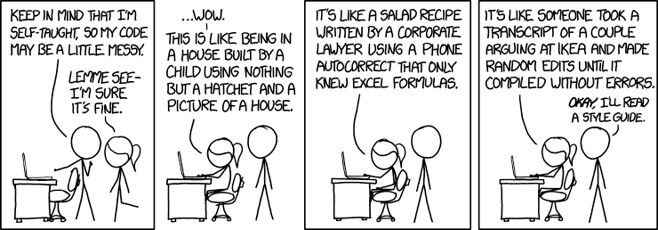
Codestyle Guidelines Bookdown
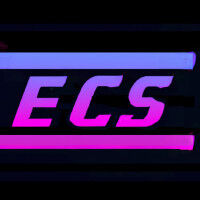
Easy Coding Standard Logo
Conclusion
The Easy Coding Standard is a valuable tool for PHP developers to improve code quality and consistency. By automating formatting tasks, you save time and can concentrate on the actual logic. The integration of ECS into your development environment and CI/CD pipeline ensures that your entire team follows the same
entire team follows the same standards and contributes to higher code quality.